Python Read Images From Mp4 in Memory
Reading and writing videos in OpenCV is very similar to reading and writing images. A video is nothing but a series of images that are ofttimes referred to equally frames. Then, all you lot demand to practise is loop over all the frames in a video sequence, and so procedure 1 frame at a time. In this post, nosotros will demonstrate how to read, display and write videos from a file, an image sequence and a webcam. We will also look into some of the errors that might occur in the procedure, and help understand how to resolve them.
- Reading videos
- From a file
- From Image-sequence
- From a webcam
- Writing videos
- Errors That One Might Face when Reading or Writing Videos
- Summary
Let'due south go through the lawmaking instance for reading a video file outset.This essentially contains the functions for reading a video from deejay and displaying it. As y'all keep further, we volition discuss the functions in particular used in this implementation.
Python
import cv2 # Create a video capture object, in this case we are reading the video from a file vid_capture = cv2.VideoCapture('Resources/Cars.mp4') if (vid_capture.isOpened() == Faux): print("Mistake opening the video file") # Read fps and frame count else: # Get frame charge per unit information # You lot tin replace five with CAP_PROP_FPS as well, they are enumerations fps = vid_capture.get(v) print('Frames per 2nd : ', fps,'FPS') # Get frame count # Yous tin replace 7 with CAP_PROP_FRAME_COUNT likewise, they are enumerations frame_count = vid_capture.go(seven) print('Frame count : ', frame_count) while(vid_capture.isOpened()): # vid_capture.read() methods returns a tuple, first chemical element is a bool # and the second is frame ret, frame = vid_capture.read() if ret == Truthful: cv2.imshow('Frame',frame) # 20 is in milliseconds, attempt to increase the value, say 50 and discover cardinal = cv2.waitKey(xx) if key == ord('q'): break else: pause # Release the video capture object vid_capture.release() cv2.destroyAllWindows()
C++
// Include Libraries #include<opencv2/opencv.hpp> #include<iostream> // Namespace to nullify use of cv::office(); syntax using namespace std; using namespace cv; int primary() { // initialize a video capture object VideoCapture vid_capture("Resources/Cars.mp4"); // Print fault message if the stream is invalid if (!vid_capture.isOpened()) { cout << "Error opening video stream or file" << endl; } else { // Obtain fps and frame count by get() method and impress // You tin can replace 5 with CAP_PROP_FPS likewise, they are enumerations int fps = vid_capture.get(five); cout << "Frames per second :" << fps; // Obtain frame_count using opencv built in frame count reading method // You can replace 7 with CAP_PROP_FRAME_COUNT too, they are enumerations int frame_count = vid_capture.get(seven); cout << " Frame count :" << frame_count; } // Read the frames to the concluding frame while (vid_capture.isOpened()) { // Initialise frame matrix Mat frame; // Initialize a boolean to check if frames are there or non bool isSuccess = vid_capture.read(frame); // If frames are present, bear witness information technology if(isSuccess == true) { //display frames imshow("Frame", frame); } // If frames are not at that place, close it if (isSuccess == false) { cout << "Video photographic camera is disconnected" << endl; break; } //wait 20 ms between successive frames and pause the loop if primal q is pressed int key = waitKey(20); if (cardinal == 'q') { cout << "q key is pressed by the user. Stopping the video" << endl; interruption; } } // Release the video capture object vid_capture.release(); destroyAllWindows(); return 0; }
NEW Form LIVE ON KICKSTARTER
Deep Learning With TensorFlow & Keras
These are the primary functions in OpenCV video I/O that we are going to discuss in this blog post:
-
cv2.VideoCapture
– Creates a video capture object, which would help stream or display the video. -
cv2.VideoWriter
– Saves the output video to a directory. - In addition, we likewise discuss other needed functions such as
cv2.imshow()
,cv2.waitKey()
and theget()
method which is used to read the video metadata such as frame height, width, fps etc.
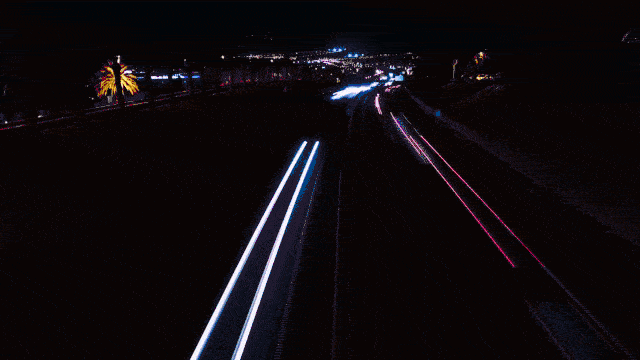
In this example, yous volition be reading the higher up video ('Cars.mp4') and displaying information technology.
We have provided the codes both in Python and C++ languages for your study and practice.
Now allow's start with it.
First nosotros import the OpenCV library. Please note that for C++, y'all would normally utilise cv::office()
, but considering we choose to use the cv
namespace (using namespace cv
), we can access the OpenCV functions direct, without pre-awaiting cv::
to the function name.
Python
# Import libraries import cv2
Download Lawmaking To easily follow along this tutorial, delight download code by clicking on the button below. It's FREE!
C++
// Include Libraries #include<opencv2/opencv.hpp> #include<iostream> using namespace std; using namespace cv;
Reading Video From a File
The next code block below uses the VideoCapture()
class to create a VideoCapture object, which we will and then employ to read the video file. The syntax for using this class is shown below:
VideoCapture(path, apiPreference)
The first argument is the filename/path to the video file. The 2d is an optional statement, indicating an API
preference. Some of the options associated with this optional argument volition be discussed further below. To learn more than most apiPreference
, visit the official documentation link VideoCaptureAPIs.
Python
# Create a video capture object, in this case nosotros are reading the video from a file vid_capture = cv2.VideoCapture('Resources/Cars.mp4')
C++
# Create a video capture object, in this example nosotros are reading the video from a file VideoCapture vid_capture("Resources/Cars.mp4");
For all the examples given below, you tin can utilise the same video file that nosotros have used here, which is available in the Downloadable Code folder, or you can use your own video file.
Now that we take a video capture object we can use the isOpened()
method to confirm the video file was opened successfully. The isOpened()
method returns a boolean that indicates whether or non the video stream is valid. Otherwise you volition go an error message. The fault message tin can imply many things. One of them is that the entire video is corrupted, or some frames are corrupted. Assuming the video file was opened successfully, nosotros tin use the get()
method to recall of import metadata associated with the video stream. Note that this method does not use to web cameras. The get()
method takes a unmarried argument from an enumerated list of options documented hither. In the instance beneath, nosotros provided the numeric values five and 7, which represent to the frame rate (CAP_PROP_FPS
) and frame count (CAP_PROP_FRAME_COUNT
). The numeric value or the name tin exist supplied.
Python
if (vid_capture.isOpened() == False): impress("Mistake opening the video file") else: # Get frame rate information fps = int(vid_capture.get(5)) print("Frame Charge per unit : ",fps,"frames per 2d") # Become frame count frame_count = vid_capture.get(7) impress("Frame count : ", frame_count)
C++
if (!vid_capture.isOpened()) { cout << "Error opening video stream or file" << endl; } else { // Obtain fps and frame count by go() method and print int fps = vid_capture.become(5): cout << "Frames per 2nd :" << fps; frame_count = vid_capture.get(7); cout << "Frame count :" << frame_count; }
After retrieving the desired metadata associated with the video file, nosotros are now ready to read each image frame from the file. This is accomplished by creating a loop and reading one frame at a time from the video stream using the vid_capture.read()
method.
The vid_capture.read()
method returns a tuple, where the first element is a boolean and the next element is the actual video frame. When the first chemical element is True, it indicates the video stream contains a frame to read.
If at that place is a frame to read, you tin can and so employ imshow()
to display the current frame in a window, otherwise get out the loop. Find that you lot also employ the waitKey()
function to pause for 20ms between video frames. Calling the waitKey()
role lets you monitor the keyboard for user input. In this example, for example, if the user presses the 'q
' fundamental, you exit the loop.
Python
while(vid_capture.isOpened()): # vCapture.read() methods returns a tuple, first element is a bool # and the second is frame ret, frame = vid_capture.read() if ret == True: cv2.imshow('Frame',frame) g = cv2.waitKey(20) # 113 is ASCII code for q primal if one thousand == 113: pause else: interruption
C++
while (vid_capture.isOpened()) { // Initialize frame matrix Mat frame; // Initialize a boolean to bank check if frames are there or not bool isSuccess = vid_capture.read(frame); // If frames are present, show it if(isSuccess == true) { //display frames imshow("Frame", frame); } // If frames are not in that location, shut it if (isSuccess == false) { cout << "Video camera is disconnected" << endl; suspension; } //look 20 ms betwixt successive frames and break the loop if key q is pressed int key = waitKey(20); if (key == 'q') { cout << "q key is pressed past the user. Stopping the video" << endl; break; } }
Once the video stream is fully processed or the user prematurely exits the loop, you release the video-capture object (vid_capture
) and shut the window, using the following code:
Python
# Release the objects vid_capture.release() cv2.destroyAllWindows()
C++
// Release video capture object vid_capture.release(); destroyAllWindows();
Reading an Image Sequence
Processing image frames from an image sequence is very similar to processing frames from a video stream. Merely specify the image files which are being read.
In the example beneath,
- You continue using a video-capture object
- Only instead of specifying a video file, you merely specify an prototype sequence
- Using the annotation shown below (Cars%04d.jpg), where %04d indicates a four-digit sequence-naming convention (e.one thousand. Cars0001.jpg, Cars0002.jpg, Cars0003.jpg, etc).
- If you had specified "Race_Cars_%02d.jpg" and so you would be looking for files of the form:
(Race_Cars_01.jpg, Race_Cars_02.jpg, Race_Cars_03.jpg, etc…).
All other codes described in the first instance would be the same.
Python
vid_capture = cv2.VideoCapture('Resources/Image_sequence/Cars%04d.jpg')
C++
VideoCapture vid_capture("Resources/Image_sequence/Cars%04d.jpg");
Reading Video from a Webcam
Reading a video stream from a web camera is also very similar to the examples discussed above. How's that possible? It's all thanks to the flexibility of the video capture class in OpenCV, which has several overloaded functions for convenience that take unlike input arguments. Rather than specifying a source location for a video file or an paradigm sequence, you simply need to give a video capture device index, as shown below.
- If your system has a congenital-in webcam, then the device index for the camera will be '
0
'. - If you accept more than than ane camera continued to your system, then the device index associated with each additional camera is incremented (eastward.1000.
1
,2
, etc).
Python
vid_capture = cv2.VideoCapture(0, cv2.CAP_DSHOW)
C++
VideoCapture vid_capture(0);
You might be wondering most the flag CAP_DSHOW
. This is an optional argument, and is therefore not required. CAP_DSHOW
is only another video-capture API preference, which is brusque for directshow via video input.
Writing Videos
Let's now take a await at how to write videos. Just like video reading, we can write videos originating from any source (a video file, an image sequence, or a webcam). To write a video file:
- Retrieve the paradigm frame height and width, using the
get()
method. - Initialize a video capture object (equally discussed in the previous sections), to read the video stream into retentivity, using whatever of the sources previously described.
- Create a video writer object.
- Apply the video author object to relieve the video stream to disk.
Continuing with our running example, let'southward kickoff by using the get()
method to obtain the video frame width and superlative.
Python
# Obtain frame size information using get() method frame_width = int(vid_capture.get(3)) frame_height = int(vid_capture.get(4)) frame_size = (frame_width,frame_height) fps = 20
C++
// Obtain frame size information using get() method Int frame_width = static_cast<int>(vid_capture.get(3)); int frame_height = static_cast<int>(vid_capture.become(four)); Size frame_size(frame_width, frame_height); int fps = 20;
Equally previously discussed, the get()
method from the VideoCapture()
class requires:
- A single statement from an enumerated list that allows you to retrieve a diverseness of metadata associated with the video frames.
The metadata bachelor is all-encompassing, and can be plant hither.
- In this example, you are retrieving the frame width and peak, past specifying
3
(CAP_PROP_FRAME_WIDTH
) and4
(CAP_PROP_FRAME_HEIGHT
). Y'all will use these dimensions further below, when writing the video file to disk.
In society to write a video file, you need to first create a video-author object from the VideoWriter()
grade, as shown in the code beneath.
Here'south the syntax for VideoWriter()
:
VideoWriter(filename, apiPreference, fourcc, fps, frameSize[, isColor])
The VideoWriter()
class takes the following arguments:
-
filename
: pathname for the output video file -
apiPreference
: API backends identifier -
fourcc
: iv-character code of codec, used to shrink the frames (fourcc) -
fps
: Frame charge per unit of the created video stream -
frame_size
: Size of the video frames -
isColor
: If not zero, the encoder will expect and encode colour frames. Else information technology will work with grayscale frames (the flag is currently supported on Windows only).
The following code creates the video author object, output
from the VideoWriter()
class. A special convenience part is used to retrieve the four-character codec, required every bit the second argument to the video writer object, cv2
.
-
VideoWriter_fourcc('M', 'J', 'P', 'G')
in Python. -
VideoWriter::fourcc('K', 'J', 'P', 'K')
in C++.
The video codec specifies how the video stream is compressed. It converts uncompressed video to a compressed format or vice versa. To create AVI or MP4 formats, employ the following fourcc specifications:
AVI: cv2.VideoWriter_fourcc('M','J','P','G')
MP4: cv2.VideoWriter_fourcc(*'XVID')
The next 2 input arguments specify the frame rate in FPS, and the frame size (width, pinnacle).
Python
# Initialize video writer object output = cv2.VideoWriter('Resources/output_video_from_file.avi', cv2.VideoWriter_fourcc('Thou','J','P','G'), 20, frame_size)
C++
//Initialize video writer object VideoWriter output("Resources/output.avi", VideoWriter::fourcc('Yard', 'J', 'P', 'G'),frames_per_second, frame_size);
Now that you lot accept created a video writer object, use it to write the video file to disk, one frame at a time, equally shown in the code below. Here, you are writing an AVI video file to disk, at 20 frames per second. Annotation how nosotros accept simplified to loop from the previous examples.
Python
while(vid_capture.isOpened()): # vid_capture.read() methods returns a tuple, first element is a bool # and the second is frame ret, frame = vid_capture.read() if ret == True: # Write the frame to the output files output.write(frame) else: impress('Stream disconnected') break
C++
while (vid_capture.isOpened()) { // Initialize frame matrix Mat frame; // Initialize a boolean to cheque if frames are there or not bool isSuccess = vid_capture.read(frame); // If frames are not there, shut it if (isSuccess == faux) { cout << "Stream disconnected" << endl; break; } // If frames are present if(isSuccess == true) { //brandish frames output.write(frame); // display frames imshow("Frame", frame); // wait for twenty ms between successive frames and break // the loop if key q is pressed int key = waitKey(20); if (key == 'q') { cout << "Central q key is pressed by the user. Stopping the video" << endl; pause; } } }
Finally, in the code below, release the video capture and video-writer objects.
Python
# Release the objects vid_capture.release() output.release()
C++
// Release the objects vid_capture.release(); output.release();
Errors That 1 Might Face up when Reading or Writing Videos
Video reading
While reading frames it can throw an error if the path is incorrect or the file is corrupted or frame is missing.That's why nosotros are using an if
statement within the while
loop. Which can be seen in the line, if ret == True
. This way information technology volition procedure it only when frames are present. Following is an example of an mistake log that is observed in this case. Information technology is non the full log, only cardinal parameters are included.
cap_gstreamer.cpp:890: error: (-two) GStreamer: unable to outset pipeline in role
For wrong path:
When you provide a incorrect path to a video, then it will not show whatever fault or alert using the VideoCapture()
grade. The issues will ascend when you will try to do any operation on the video frames. For this, you can use a uncomplicated if cake for checking whether you have read a video file or not like nosotros did in our example. That should impress the following bulletin.
Fault opening the video file
Video writing
In this step various errors can occur. About mutual are frame size mistake and api preference mistake. If the frame size is not similar to the video, and so fifty-fifty though nosotros get a video file at the output directory, it will be bare. If you are using the NumPy shape method to recollect frame size, remember to reverse the output as OpenCV will return tiptop x width ten channels . If it is throwing an api preference error, we might need to pass the CAP_ANY
flag in the VideoCapture()
argument. It can be seen in the webcam example, where we are using CAP_DHOW
to avoid warnings being generated.
Following are the examples of error logs:
When CAP_DSHOW is non passed :
[WARN:0]...cap_msmf.cpp(438) …. terminating async callback
When frame size is not correct:
cv2.error: OpenCV(4.five.2) :-1: error: (-5:Bad statement) in function 'VideoWriter'
> Overload resolution failed:
> - Can't parse 'frameSize'. Sequence particular with index 0 has a wrong type
> - VideoWriter() missing required statement 'frameSize' (pos 5)
> - VideoWriter() missing required argument 'params' (pos 5)
> - VideoWriter() missing required argument 'frameSize' (pos 5)
Expand Your Knowledge
NEW COURSE LIVE ON KICKSTARTER
Deep Learning With TensorFlow & Keras
Summary
In this blog, you learned to read and brandish video streams from three different sources, using a video-capture object. Even saw how to use a video-capture object to recall important metadata from the video stream. We also demonstrated how to write video streams to disk, using a video-writer object. You may besides find it helpful to resize video frames, or annotate them with shapes and text. For this, just modify the individual image frames.
At present that y'all know how to read and write videos, and are comfortable using OpenCV,keep up the footstep. And go on learning.
Subscribe & Download Lawmaking
Subscribe & Download Lawmaking
If you liked this article and would like to download lawmaking (C++ and Python) and case images used in this post, delight click hither. Alternately, sign up to receive a free Computer Vision Resource Guide. In our newsletter, nosotros share OpenCV tutorials and examples written in C++/Python, and Computer Vision and Car Learning algorithms and news.
Python Read Images From Mp4 in Memory
Source: https://learnopencv.com/reading-and-writing-videos-using-opencv/
- Dapatkan link
- X
- Aplikasi Lainnya
Komentar
Posting Komentar